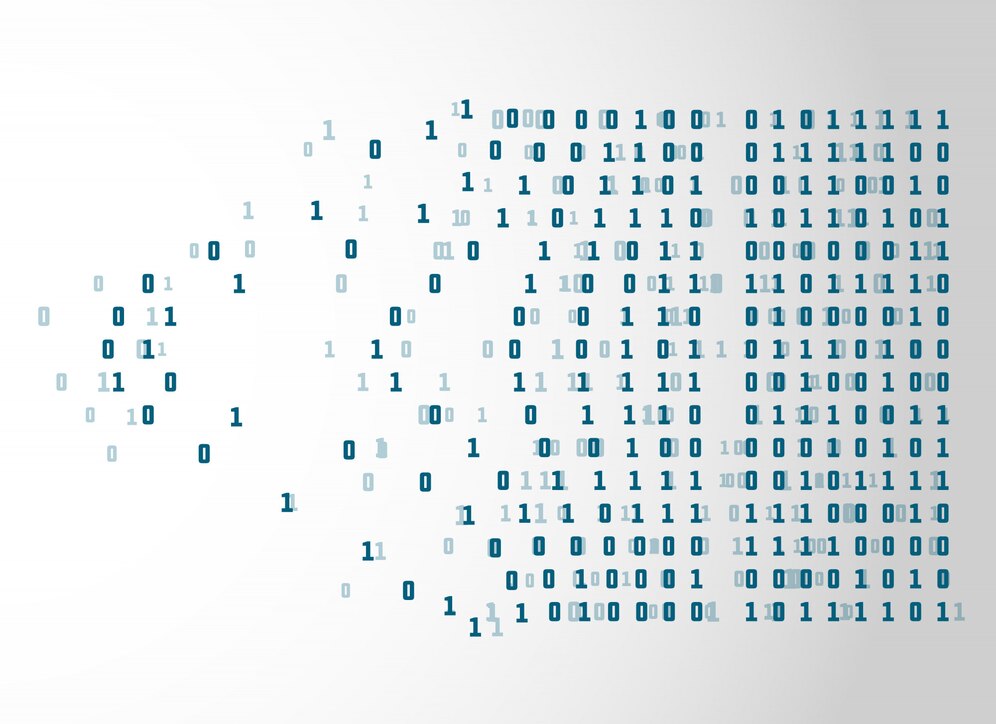
Photo by starline
Here’s a simple Python program to demonstrate reading from and writing to binary files:
Writing to a Binary File:
def write_binary_file(filename, data):
with open(filename, 'wb') as file: # 'wb' mode for writing binary
file.write(data)
print(f"Data written to {filename} successfully.")
Reading from a Binary File:
def read_binary_file(filename):
with open(filename, 'rb') as file: # 'rb' mode for reading binary
data = file.read()
print(f"Data read from {filename}: {data}")
return data
Example usage:
# Data to be written (can be text converted to bytes or any binary data)
data_to_write = b'This is some binary data.'
# Writing the data to 'example.bin'
write_binary_file('example.bin', data_to_write)
# Reading the data from 'example.bin'
read_binary_file('example.bin')
Explanation:
- wb: Opens the file in binary write mode. If the file doesn’t exist, it creates one. If it exists, it overwrites the content.
- rb: Opens the file in binary read mode. This reads raw binary data.
- The data written is in byte format (b’…’).
This program will write the binary data to a file named example.bin and then read it back to verify the contents.
Python Code Example for Writing and Reading Complex Binary Data:
1. Writing a Binary File (with structured data)
We’ll use Python’s struct module to encode various types of data (like integers, floats, etc.) into binary format. Then, we’ll write this binary data into a file.
import struct
def write_complex_binary_file(filename):
with open(filename, 'wb') as file:
# Write an integer (4 bytes), a float (4 bytes), and a string (in bytes).
integer_value = 2024
float_value = 99.99
string_value = "HBSS Academy"
# Pack the integer and float into binary format
binary_data = struct.pack('if', integer_value, float_value) # 'i' for int, 'f' for float
# Write binary data to the file
file.write(binary_data)
# Now, let's write the string in binary format
encoded_string = string_value.encode('utf-8') # Convert string to bytes
file.write(encoded_string)
print(f"Data written to {filename} successfully.")
# Example usage:
write_complex_binary_file(‘complex_data.bin’)
2. Reading a Binary File
We need to read the binary data back and decode it into the original types.
def read_complex_binary_file(filename):
with open(filename, 'rb') as file:
# Read 8 bytes: 4 for the integer and 4 for the float
binary_data = file.read(8) # First 8 bytes contain integer and float
# Unpack the binary data
integer_value, float_value = struct.unpack('if', binary_data) # 'i' for int, 'f' for float
print(f"Integer value: {integer_value}")
print(f"Float value: {float_value}")
# Read the remaining bytes for the string (assume we know the string length)
remaining_data = file.read() # Read till the end of the file
# Decode the remaining binary data as a UTF-8 string
string_value = remaining_data.decode('utf-8')
print(f"String value: {string_value}")
# Example usage:
read_complex_binary_file('complex_data.bin')
Detailed Explanation:
Step 1: Writing to the Binary File
- We use the struct module to pack different data types (like an integer and a float) into binary format. The format specifiers:
- ‘i’ stands for a 4-byte integer.
- ‘f’ stands for a 4-byte float.
- After packing the integer and float, we directly write the bytes to the file using file.write().
- Then we encode the string “HBSS Academy” into bytes using utf-8 encoding and write that to the file as well.
Step 2: Reading from the Binary File
- When reading the file, we first read 8 bytes (4 for the integer and 4 for the float) and unpack them using struct.unpack().
- After that, we read the rest of the file, assuming it’s the string. We decode these bytes back into a string using utf-8 decoding.
Also Learn Develop a Log Parser to Analyze Server Logs and Extract Meaningful Insights in Python
Output:
When running the write and read operations, the output would look something like this:
Data written to complex_data.bin successfully.
# Reading data from file:
Integer value: 2024
Float value: 99.98999786376953 # (close to 99.99, slight precision loss due to binary format)
String value: HBSS Academy
Example 2: Writing and Reading an Image as Binary Data
Writing Image Data:
def write_image_binary(input_image, output_filename):
with open(input_image, 'rb') as img_file:
binary_data = img_file.read() # Read entire image as binary data
with open(output_filename, 'wb') as output_file:
output_file.write(binary_data)
print(f"Image binary data written to {output_filename}")
# Example usage:
write_image_binary('input_image.jpg', 'output_image.bin')
Reading the Binary File as an Image:
def read_image_binary(binary_filename, output_image):
with open(binary_filename, 'rb') as binary_file:
binary_data = binary_file.read() # Read binary data from file
with open(output_image, 'wb') as img_file:
img_file.write(binary_data) # Write binary data back to a new image file
print(f"Binary data restored as image: {output_image}")
# Example usage:
read_image_binary('output_image.bin', 'restored_image.jpg')
Explanation:
- In the image example, the binary data of the image is directly read as bytes and written to a file. Then, it can be restored from the binary file back into an image.
This approach can handle larger files, such as media, while the first example demonstrates working with structured binary data.
Leave a Reply