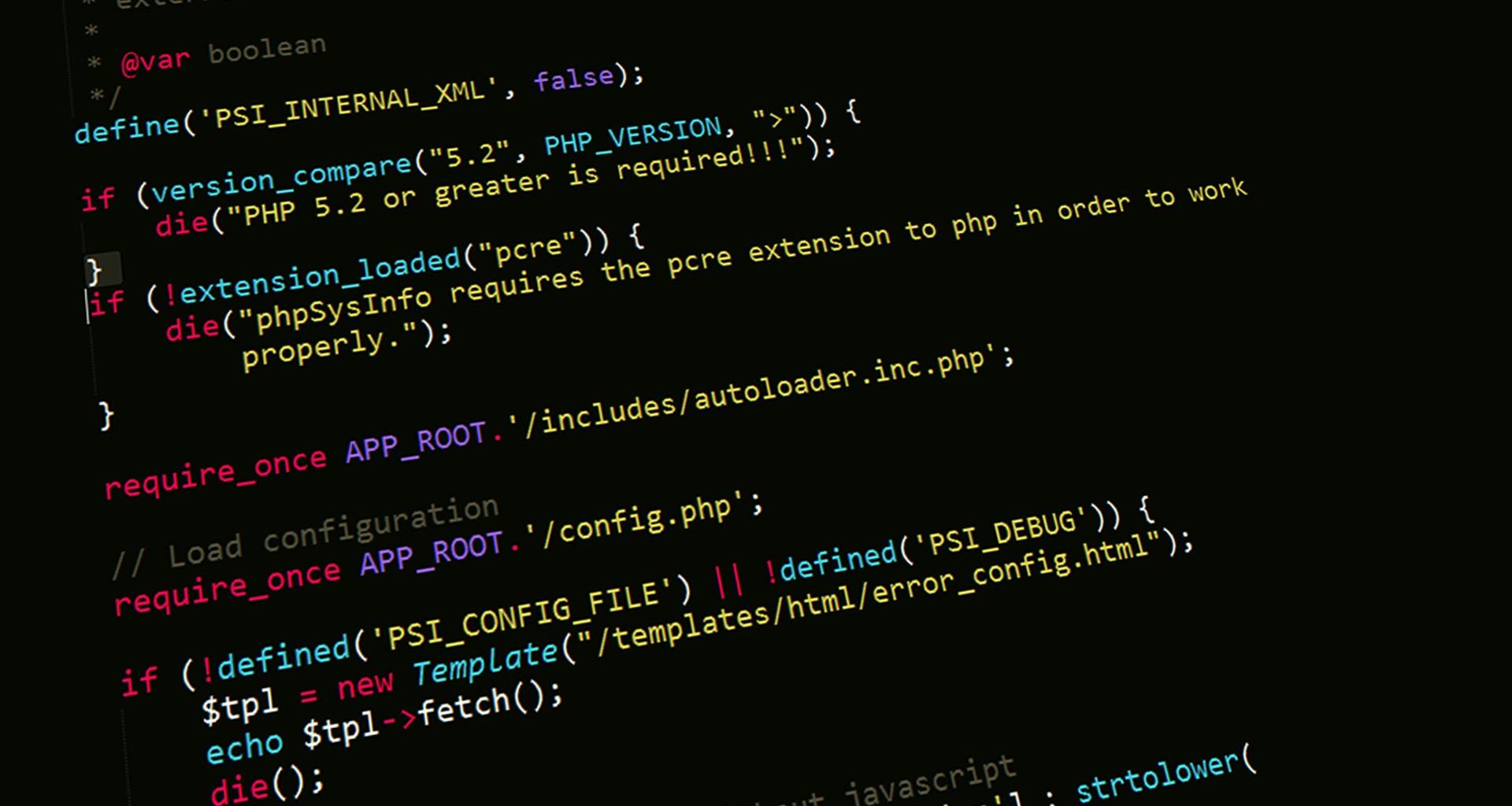
Photo by Pixabay
A fruitful function in Python is a function that returns a value after executing its code. These functions are called “fruitful” because they produce or return something, unlike void functions which don’t return a value (they return None by default).
Here’s an example of a fruitful function in Python:
Example: Sum of Two Numbers
def add_numbers(a, b):
"""This function takes two numbers and returns their sum."""
result = a + b
return result
# Using the fruitful function
sum_result = add_numbers(5, 7)
print("The sum is:", sum_result)
Explanation:
- Function Definition: The function add_numbers takes two parameters, a and b.
- Computation: Inside the function, the sum of a and b is calculated and stored in the variable result.
- Return Statement: The return statement sends the value of result back to the caller of the function.
- Using the Function: When we call add_numbers(5, 7), it returns the sum of 5 and 7, which is then printed as “The sum is: 12”.
Example: Check if a Number is Even
Here’s another example where the function checks if a number is even:
def is_even(number):
"""This function returns True if the number is even, False otherwise."""
return number % 2 == 0
# Using the fruitful function
num = 10
if is_even(num):
print(f"{num} is even.")
else:
print(f"{num} is odd.")
Explanation:
- Function Definition: The function is_even takes one parameter number.
- Return Statement: It returns True if the number is even (number % 2 == 0), and False otherwise.
- Using the Function: The is_even(10) call returns True, so the output is “10 is even.”
Summary
- A fruitful function returns a value.
- Use the return statement to send a value back to the caller.
- Examples include functions that perform calculations, checks, or any operations that yield a result.
These functions are essential for writing reusable, modular code in Python.
Leave a Reply