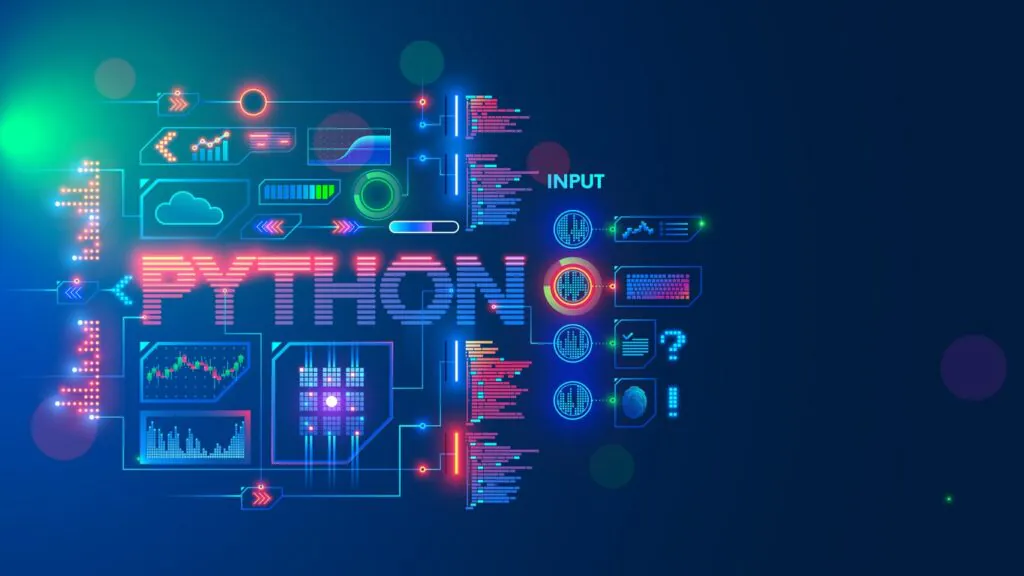
Photo by CODEMOTION
Recentering a matrix typically means adjusting the matrix so that its mean is centered around a specific value, usually zero. This is often done as a preprocessing step in machine learning and data analysis to improve numerical stability and model performance.
Steps to Recentering a Matrix
- Calculate the Mean of Each Column: Compute the mean of each column in the matrix.
- Subtract the Mean: Subtract the mean from each element in the corresponding column.
Here’s how you can perform these steps in Python using libraries like NumPy:
Example Code
import numpy as np
# Sample matrix
matrix = np.array([
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
])
print("Original Matrix:")
print(matrix)
# Step 1: Calculate the mean of each column
mean = np.mean(matrix, axis=0)
# Step 2: Subtract the mean from each element in the corresponding column
recentered_matrix = matrix - mean
print("\nRecentered Matrix:")
print(recentered_matrix)
Explanation
- np.mean(matrix, axis=0): Computes the mean of each column (axis=0 specifies column-wise operation).
- matrix – mean: Subtracts the mean from each element in the corresponding column.
Output
Original Matrix:
[[1 2 3]
[4 5 6]
[7 8 9]]
Recentered Matrix:
[[-3. -3. -3.]
[ 0. 0. 0.]
[ 3. 3. 3.]]
Additional Considerations
- Recenter by Rows: If you need to center by rows instead of columns, use axis=1 in the np.mean() function:
mean = np.mean(matrix, axis=1, keepdims=True)
recentered_matrix = matrix - mean
- Mean Centering for a 2D Matrix: The above example shows centering each column. If your application requires different methods, such as centering the entire matrix (subtracting the global mean from all elements), you would adjust the code accordingly.
Summary
Recentering a matrix involves subtracting the mean of each column from the matrix’s elements, which is a common preprocessing step to ensure that the data is centred around zero. This technique can be applied to data matrices for better performance in various data analysis and machine learning tasks.
Leave a Reply