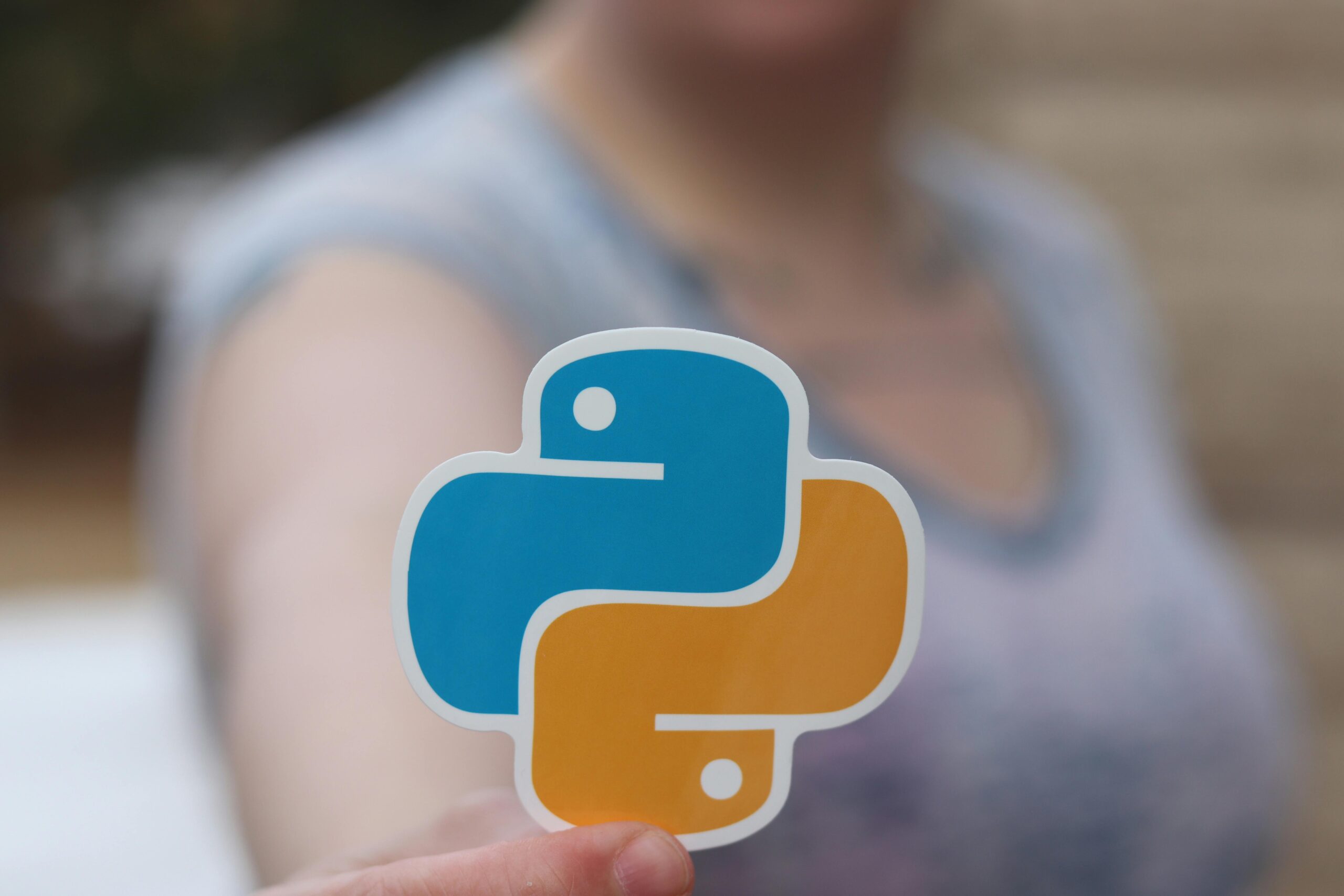
Photo by RealToughCandy.com
The code below is a python code to add two numbers and display the output.
# Function to add two numbers
def add_numbers(number1, number2):
return number1 + number2
# Prompt the user to enter the first number
number1 = float(input("Enter the first number: "))
# Prompt the user to enter the second number
number2 = float(input("Enter the second number: "))
# Call the function to add the numbers
sum = add_numbers(number1, number2)
# Display the sum
print("The sum of", number1, "and", number2, "is", sum)
Explanation:
1. Define a function: add_numbers is a function that accepts two arguments and returns their sum.
3. Call the function: Using the two input numbers, we call the add_numbers function and save the outcome in the variable total. 4. Present the outcome: Lastly, we use the print function to output the total. You will be prompted to enter two numbers, and the program will display their sum.def add_numbers(number1, number2):
return number1 + number2
number1 = float(input("Enter the first number: "))
number2 = float(input("Enter the second number: "))
sum = add_numbers(number1, number2)
print("The sum of", number1, "and", number2, "is", sum)
How to Run the Program:
python add_numbers.py
Leave a Reply