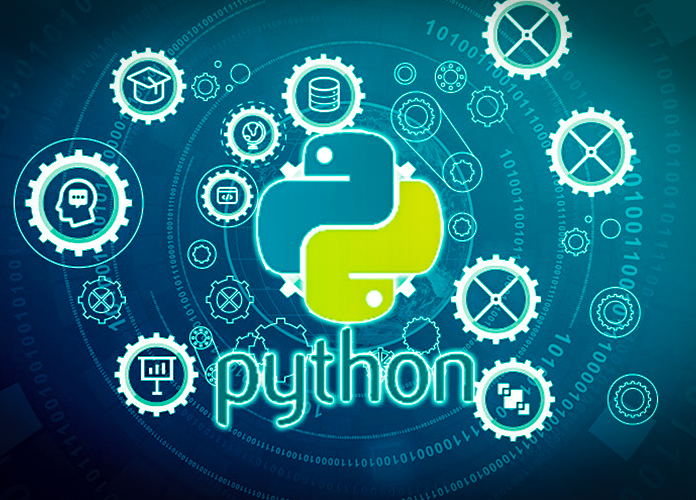
Photo by Eduonix
NMADS stands for “Neural-Masked Average Distribution Sampling”, which is a method related to machine learning and neural networks. NMADS is designed to improve sampling efficiency and accuracy in various machine learning tasks, especially when working with large and complex datasets.
If you’re looking for Python implementations or applications of NMADS, it might be useful in contexts like generative models or neural network training. Here’s a general overview of how such techniques might be implemented in Python, though specific implementations can vary based on the exact application:
General Approach for NMADS in Python
- Define Neural Network Model:
- Use libraries like TensorFlow or PyTorch to define your neural network model. This will be the base model from which you will perform sampling.
- Implement Sampling Strategy:
- Define how the NMADS method will sample from the model’s distribution. This often involves defining a masked sampling mechanism that adjusts based on the network’s outputs.
- Train the Model:
- Train your neural network using typical training procedures, integrating NMADS as part of your data sampling strategy or loss function.
- Evaluate and Adjust:
- Evaluate the performance of your model and the effectiveness of the NMADS strategy, making adjustments as necessary.
Example Code Snippet (Conceptual)
Here’s a simplified example using PyTorch to give an idea of how sampling might be integrated into a neural network workflow:
import torch
import torch.nn as nn
import torch.optim as optim
# Define a simple neural network
class SimpleNN(nn.Module):
def __init__(self):
super(SimpleNN, self).__init__()
self.fc1 = nn.Linear(10, 50)
self.fc2 = nn.Linear(50, 2) # Output layer for binary classification
def forward(self, x):
x = torch.relu(self.fc1(x))
x = self.fc2(x)
return x
# Initialize model, loss function, and optimizer
model = SimpleNN()
criterion = nn.CrossEntropyLoss()
optimizer = optim.Adam(model.parameters(), lr=0.001)
# Training loop (simplified)
for epoch in range(10): # Example epoch count
optimizer.zero_grad()
# Dummy input and target
inputs = torch.randn(64, 10) # Batch of 64 samples, each with 10 features
targets = torch.randint(0, 2, (64,)) # Binary targets
# Forward pass
outputs = model(inputs)
# Compute loss
loss = criterion(outputs, targets)
# Backward pass and optimization
loss.backward()
optimizer.step()
print(f'Epoch {epoch+1}, Loss: {loss.item()}')
# Define NMADS-specific sampling here
# This could involve creating a custom sampling function based on model outputs
def nmads_sampling(outputs):
# Example of a masked sampling function (conceptual)
probabilities = torch.softmax(outputs, dim=1)
# Apply some masking strategy here
sampled_indices = torch.multinomial(probabilities, 1)
return sampled_indices
# Evaluate using NMADS sampling
outputs = model(torch.randn(1, 10))
sampled = nmads_sampling(outputs)
print(f'Sampled index: {sampled.item()}')
Summary
- NMADS: A method used to improve sampling efficiency in machine learning.
- Implementation: Typically involves defining a neural network, implementing a sampling strategy, and integrating it into your training and evaluation processes.
For specific implementations or papers detailing NMADS, you might need to consult specialized literature or resources related to neural network sampling techniques. If you have a more specific application or implementation in mind, please let me know!
Leave a Reply