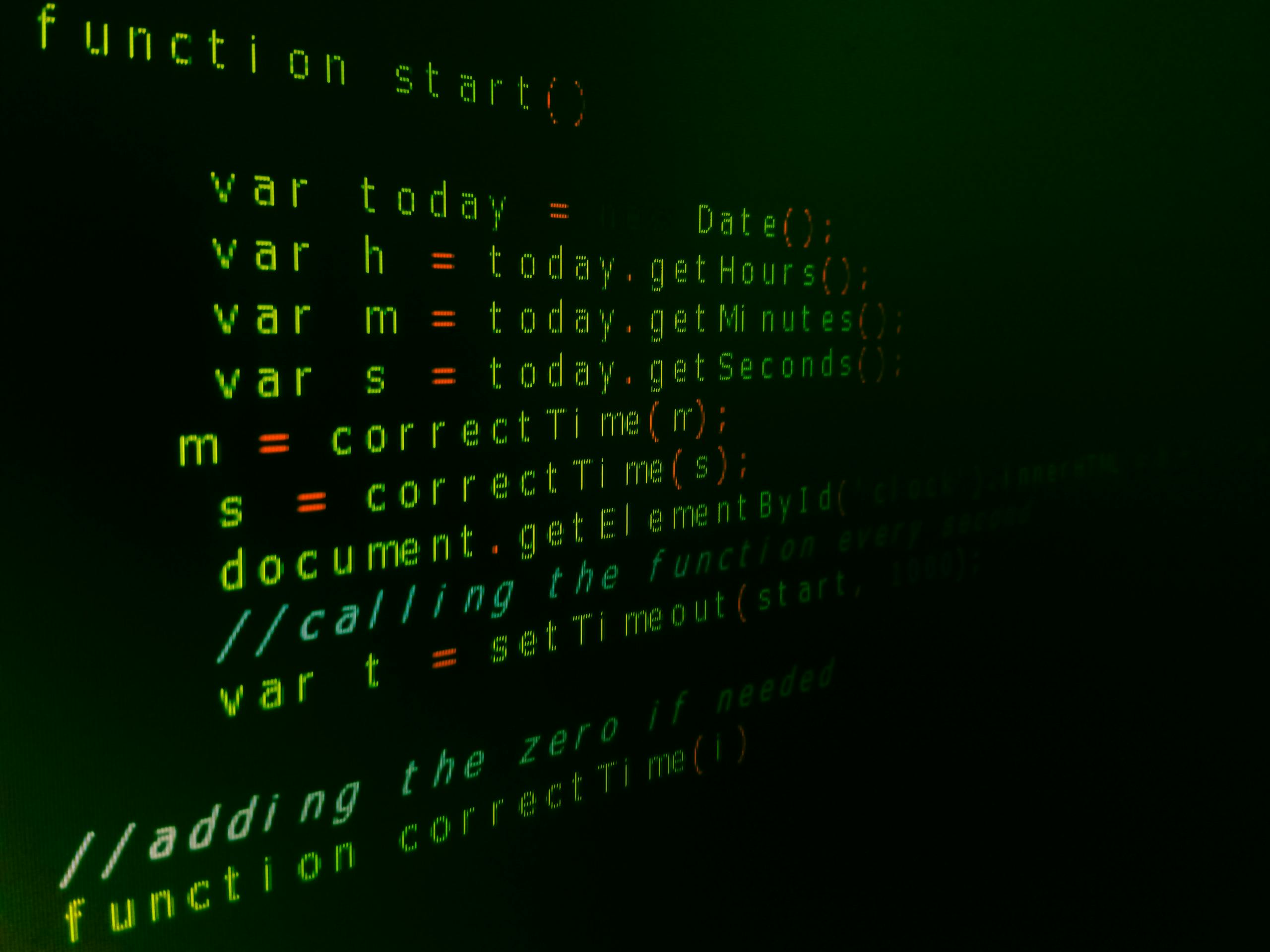
Photo by Jorge Jesus
This simple C++ program will add two numbers and display the sum.
#include <iostream>
Using namespace std;
int main() {
// Declare variables to store the numbers
double num1, num2, sum;
//Ask user to enter first number
cout << "Enter 1st number: ";
cin >> num1;
// Ask user to enter second number
cout << "Enter 2nd number: ";
cin >> num2;
// Add the two numbers
sum = num1 + num2;
// Display the sum
cout << "The sum of " << num1 << " and " << num2 << " is " << sum << endl;
return 0;
}
Explanation:
Include the iostream library: This library is used for input and output operations in C++.
#include <iostream>
Main function: The execution of a C++ program begins with the main function.
int main() {
// Code here
return 0;
}
Declare variables: We declare three variables num1, num2, and sum to store the numbers input by the user and their sum.
double num1, num2, sum;
Prompt the user for input: Using cout, we display a message to the user asking them to input two numbers. We then use cin to read the numbers.
cout << "Enter the first number: ";
cin >> num1;
cout << "Enter the second number: ";
cin >> num2;
Calculate the sum: We add num1 and num2 and store the result in the variable sum.
sum = num1 + num2;
Display the result: Finally, we output the sum using cout.
cout << "The sum of " << num1 << " and " << num2 << " is " << sum << endl;
How to Compile and Run the Program:
-
- Save the code in a file with a .cpp extension, for example, add_numbers.cpp.
-
- Open a terminal or command prompt.
-
- Navigate to the directory where you saved the file.
-
- Compile the program using a C++ compiler. For example, if you are using g++, you can compile it with the following command:
g++ -o add_numbers add_numbers.cpp
-
- Run the compiled program:
./add_numbers
You will be prompted to enter two numbers, and the program will display their sum.
Leave a Reply