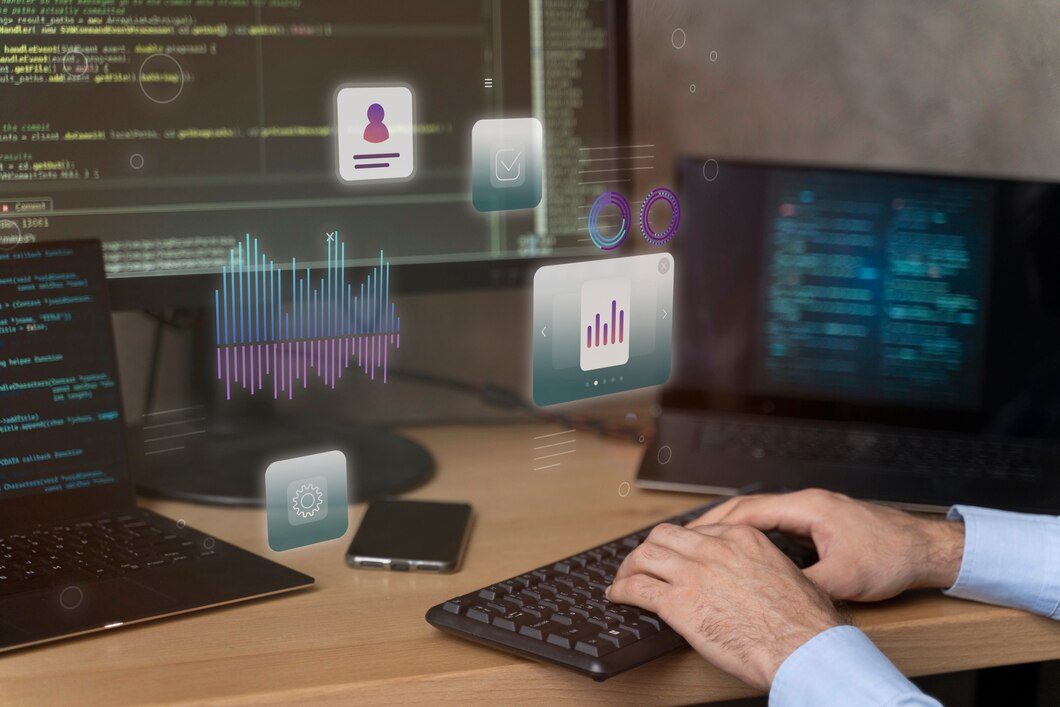
Photo by freepik
To develop a program that converts data between different formats (e.g., CSV to JSON, XML to JSON, etc.), you can leverage Python’s built-in libraries such as csv, json, and xml.etree.ElementTree. Below is a Python program that provides conversion functions between CSV, JSON, and XML formats.
Steps:
- CSV to JSON: Convert tabular data from CSV to JSON format.
- JSON to CSV: Convert structured JSON data to CSV format.
- XML to JSON: Convert hierarchical XML data to JSON format.
- JSON to XML: Convert JSON back into XML.
Full Python Script:
import csv
import json
import xml.etree.ElementTree as ET
from xml.dom import minidom
# Function to convert CSV to JSON
def csv_to_json(csv_file, json_file):
data = []
with open(csv_file, mode='r') as file:
csv_reader = csv.DictReader(file)
for row in csv_reader:
data.append(row)
with open(json_file, mode='w') as file:
json.dump(data, file, indent=4)
print(f"CSV file '{csv_file}' converted to JSON and saved as '{json_file}'.")
# Function to convert JSON to CSV
def json_to_csv(json_file, csv_file):
with open(json_file, mode='r') as file:
data = json.load(file)
with open(csv_file, mode='w', newline='') as file:
if len(data) > 0:
csv_writer = csv.DictWriter(file, fieldnames=data[0].keys())
csv_writer.writeheader()
csv_writer.writerows(data)
print(f"JSON file '{json_file}' converted to CSV and saved as '{csv_file}'.")
# Function to convert XML to JSON
def xml_to_json(xml_file, json_file):
tree = ET.parse(xml_file)
root = tree.getroot()
def parse_element(element):
parsed_data = {}
if element.text is not None and element.text.strip():
parsed_data['text'] = element.text.strip()
for child in element:
child_data = parse_element(child)
if child.tag in parsed_data:
if isinstance(parsed_data[child.tag], list):
parsed_data[child.tag].append(child_data)
else:
parsed_data[child.tag] = [parsed_data[child.tag], child_data]
else:
parsed_data[child.tag] = child_data
return parsed_data
json_data = {root.tag: parse_element(root)}
with open(json_file, mode='w') as file:
json.dump(json_data, file, indent=4)
print(f"XML file '{xml_file}' converted to JSON and saved as '{json_file}'.")
# Function to convert JSON to XML
def json_to_xml(json_file, xml_file):
with open(json_file, mode='r') as file:
data = json.load(file)
def build_xml_element(data, parent):
if isinstance(data, dict):
for key, value in data.items():
child = ET.SubElement(parent, key)
build_xml_element(value, child)
elif isinstance(data, list):
for item in data:
build_xml_element(item, parent)
else:
parent.text = str(data)
root_key = list(data.keys())[0]
root_element = ET.Element(root_key)
build_xml_element(data[root_key], root_element)
# Pretty formatting for XML
xml_str = ET.tostring(root_element, encoding='unicode')
pretty_xml_str = minidom.parseString(xml_str).toprettyxml(indent=" ")
with open(xml_file, mode='w') as file:
file.write(pretty_xml_str)
print(f"JSON file '{json_file}' converted to XML and saved as '{xml_file}'.")
# Example usage of functions
csv_file_path = 'data.csv'
json_file_path = 'data.json'
xml_file_path = 'data.xml'
# CSV to JSON conversion
csv_to_json(csv_file_path, json_file_path)
# JSON to CSV conversion
json_to_csv(json_file_path, 'output.csv')
# XML to JSON conversion
xml_to_json(xml_file_path, 'output.json')
# JSON to XML conversion
json_to_xml(json_file_path, 'output.xml')
Explanation:
1. CSV to JSON Conversion:
- csv.DictReader() reads the CSV file and converts each row into a Python dictionary.
- The rows are appended to a list, which is then saved as a JSON file using json.dump().
2. JSON to CSV Conversion:
- json.load() reads the JSON file into a Python dictionary (or list of dictionaries).
- csv.DictWriter() is used to write the JSON data into a CSV file with headers.
3. XML to JSON Conversion:
- The xml.etree.ElementTree library parses the XML into a tree structure.
- The script recursively traverses through each element, converting it into a nested Python dictionary, which is then saved as JSON.
4. JSON to XML Conversion:
- The script recursively builds XML elements from the JSON dictionary using xml.etree.ElementTree.
- The XML string is beautified using minidom.parseString() for readable formatting before saving it to the XML file.
Example Input and Output:
1. CSV File (data.csv):
name,age,city
John,25,New York
Jane,30,Los Angeles
2. JSON Output (data.json from CSV):
[
{
"name": "John",
"age": "25",
"city": "New York"
},
{
"name": "Jane",
"age": "30",
"city": "Los Angeles"
}
]
3. XML File (data.xml):
<people>
<person>
<name>John</name>
<age>25</age>
<city>New York</city>
</person>
<person>
<name>Jane</name>
<age>30</age>
<city>Los Angeles</city>
</person>
</people>
4. JSON Output (output.json from XML):
{
"people": {
"person": [
{
"name": {
"text": "John"
},
"age": {
"text": "25"
},
"city": {
"text": "New York"
}
},
{
"name": {
"text": "Jane"
},
"age": {
"text": "30"
},
"city": {
"text": "Los Angeles"
}
}
]
}
}
Customizations You Can Add:
- Error Handling: Add checks to ensure input files exist and handle different edge cases.
- User Interface: You can integrate this script into a graphical user interface (GUI) or a web app.
- File Format Detection: Automatically detect file format based on file extensions and convert accordingly.
Conclusion:
This Python program simplifies the task of converting data between formats like CSV, JSON, and XML. You can easily extend the script to handle more formats or add customizations such as selective field conversion or formatting adjustments.
Leave a Reply