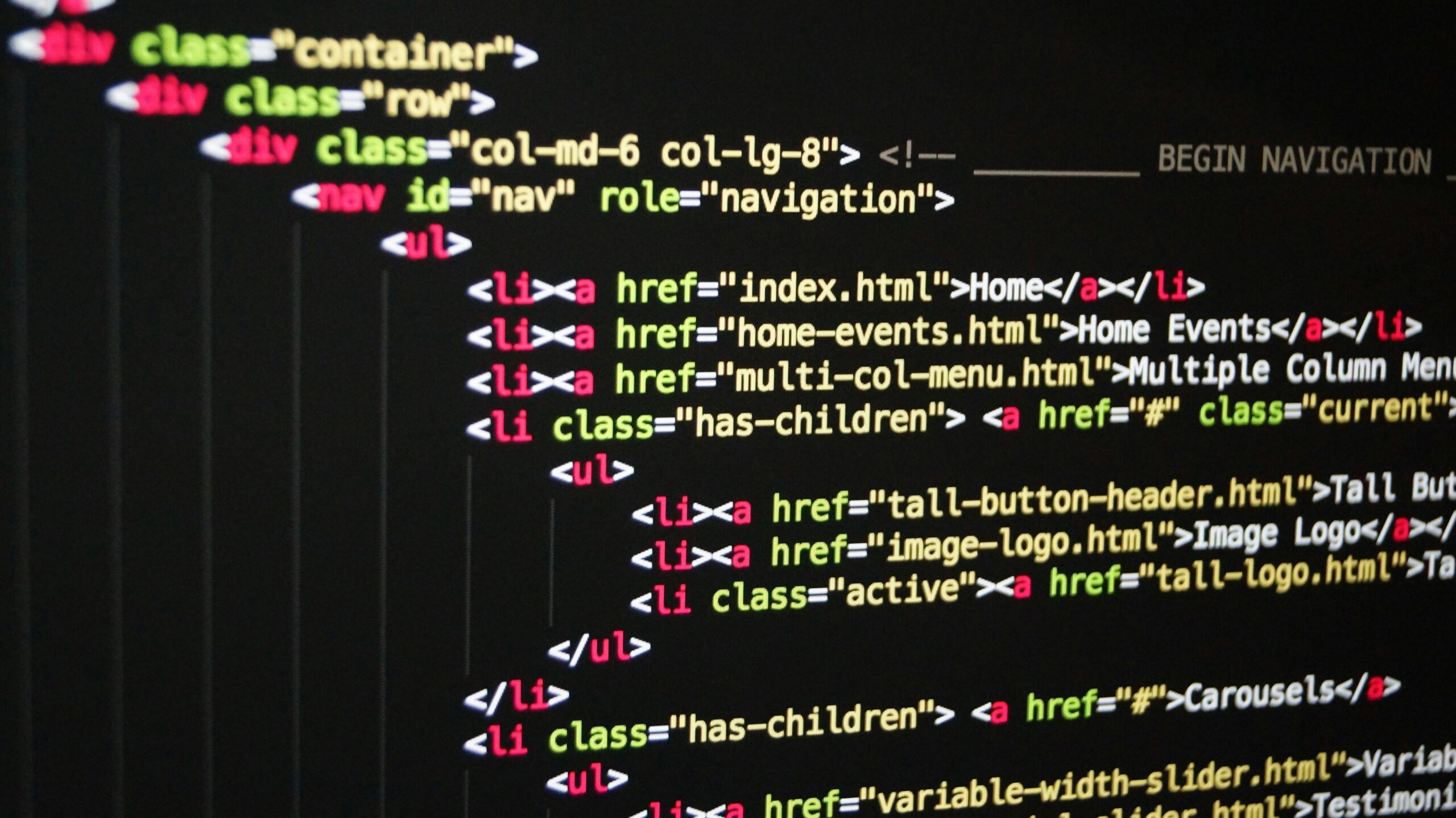
The Knapsack problem is a classic optimization problem where the goal is to determine the most valuable combination of items to include in a knapsack without exceeding its weight capacity. Dynamic programming provides an efficient way to solve this problem by breaking it down into smaller subproblems and building up the solution.
Problem Statement:
Given a list of items, each with a weight and a value, determine the maximum value that can be accumulated in a knapsack of a given capacity.
Dynamic Programming Approach:
def knapsack(weights, values, capacity):
# Number of items
n = len(weights)
# Create a DP table where dp[i][w] represents the maximum value that can be achieved with the first i items and capacity w
dp = [[0 for _ in range(capacity + 1)] for _ in range(n + 1)]
# Build the DP table
for i in range(1, n + 1):
for w in range(1, capacity + 1):
if weights[i-1] <= w:
# Either include the current item or exclude it
dp[i][w] = max(dp[i-1][w], dp[i-1][w-weights[i-1]] + values[i-1])
else:
# Exclude the current item
dp[i][w] = dp[i-1][w]
# The bottom-right corner will have the answer to the problem
return dp[n][capacity]
def main():
# Example item weights and values
weights = [2, 3, 4, 5] # Weights of the items
values = [3, 4, 5, 6] # Values of the items
capacity = 8 # Capacity of the knapsack
# Call the knapsack function
max_value = knapsack(weights, values, capacity)
print("Maximum value that can be carried:", max_value)
if __name__ == "__main__":
main()
Explanation:
- DP Table Initialization:
- dp[i][w] represents the maximum value that can be achieved with the first i items and a knapsack of capacity w.
- The DP table is initialized with zeros, where dp[i][w] = 0 when either no items are considered or the knapsack capacity is zero.
- Filling the DP Table:
- For each item i and each possible capacity w, the algorithm checks whether the current item can be included in the knapsack (weights[i-1] <= w).
- If the item can be included, the algorithm chooses the maximum value between including the item (dp[i-1][w-weights[i-1]] + values[i-1]) and not including it (dp[i-1][w]).
- If the item cannot be included, it simply carries forward the value from the previous row (dp[i-1][w]).
- Final Solution:
- The value in the bottom-right corner of the DP table (dp[n][capacity]) gives the maximum value that can be carried in the knapsack with the given capacity.
Example Output:
Maximum value that can be carried: 10
In this example:
- The items have weights [2, 3, 4, 5] and corresponding values [3, 4, 5, 6].
- With a knapsack capacity of 8, the algorithm determines that the maximum value that can be carried is 10 by selecting items with weights 3 and 5 (values 4 and 6).
Time Complexity:
- The time complexity is O(n * capacity), where n is the number of items and capacity is the capacity of the knapsack.
- The space complexity is also O(n * capacity) due to the DP table.
This dynamic programming approach is efficient and provides an optimal solution to the 0/1 Knapsack problem.
Leave a Reply