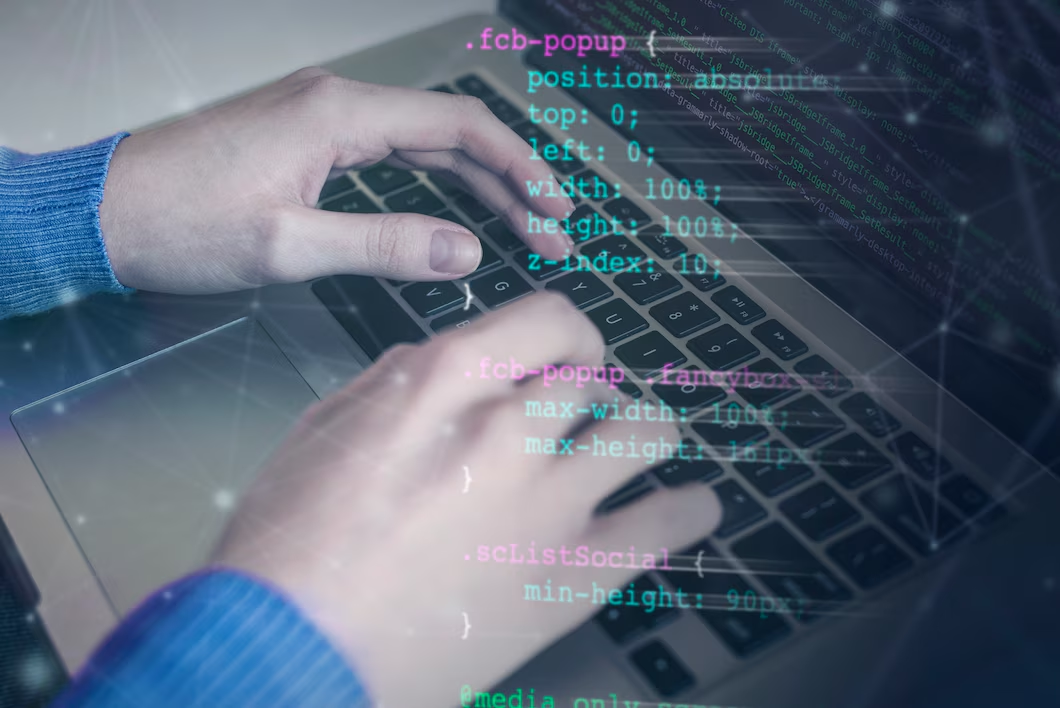
Photo by freepik
Here’s a simple Python program that allows you to rename files in bulk using a specific pattern.
import os
def bulk_rename_files(directory, pattern, start_index=1, extension=None, include_extension_in_pattern=False, exclude_files=None):
"""
Rename all files in a given directory based on a specified pattern.
Parameters:
- directory (str): The path of the directory containing the files to rename.
- pattern (str): The custom pattern to use for renaming files.
- start_index (int): Starting number for file numbering (default is 1).
- extension (str): The file extension for new filenames (None keeps original extension).
- include_extension_in_pattern (bool): If True, includes the file's original extension in the renamed file.
- exclude_files (list): A list of filenames to exclude from renaming.
"""
# List all files in the directory
files = [f for f in os.listdir(directory) if os.path.isfile(os.path.join(directory, f))]
# Filter out excluded files if specified
if exclude_files:
files = [f for f in files if f not in exclude_files]
# Iterate over each file and rename it based on the pattern
for index, filename in enumerate(files, start=start_index):
# Get file extension if not provided
original_extension = os.path.splitext(filename)[1] if not extension else extension
new_extension = original_extension if include_extension_in_pattern else extension or original_extension
# Construct the new filename
new_name = f"{pattern}_{index}{new_extension}"
old_file = os.path.join(directory, filename)
new_file = os.path.join(directory, new_name)
# Rename the file
os.rename(old_file, new_file)
print(f"Renamed: {filename} -> {new_name}")
# Parameters
directory_path = './files_to_rename' # Directory containing the files to rename
rename_pattern = 'document' # Pattern to rename files
start_number = 1 # Starting index for renaming
new_extension = '.txt' # New file extension (set to None to keep original)
include_original_extension = False # Whether to keep the original file extension in the new filename
exclude_list = ['readme.md'] # List of files to exclude from renaming
# Call the function
bulk_rename_files(directory_path, rename_pattern, start_number, new_extension, include_original_extension, exclude_list)
Program Details
- Parameters:
- directory: Path to the directory containing files to rename.
- pattern: The base name for renaming. For example, “document”.
- start_index: You can start numbering from any number (default is 1).
- extension: Specify a new file extension (e.g., .txt). If set to None, it will keep the original extension.
- include_extension_in_pattern: If True, the original file extension will be included in the new filename (e.g., document_1.csv).
- exclude_files: A list of files that should not be renamed (e.g., [‘readme.md’]).
- New Features:
- Flexible Extension Handling: You can either assign a new extension to renamed files or keep their original extensions.
- File Exclusion: Exclude specific files from renaming by providing a list of filenames (e.g., [‘readme.txt’]).
- Pattern Flexibility: The program adds an index number to the specified pattern (e.g., document_1, document_2).
Example Scenarios:
1. Simple Renaming with a New Pattern
You have a folder with:
- fileA.txt
- fileB.pdf
- fileC.docx
Using the following parameters:
- pattern = ‘report’
- extension = None
The renamed files will become:
- report_1.txt
- report_2.pdf
- report_3.docx
2. Renaming Files with a New Extension
You have a folder with:
- image1.png
- image2.jpg
Using the following parameters:
- pattern = ‘photo’
- extension = ‘.jpg’
The renamed files will become:
- photo_1.jpg
- photo_2.jpg
3. Excluding Specific Files
You have a folder with:
- doc1.txt
- doc2.txt
- readme.md
Using the following parameters:
- exclude_files = [‘readme.md’]
The renamed files will become:
- document_1.txt
- document_2.txt
- readme.md (unchanged)
4. Including Original Extension in the Pattern
You have a folder with:
- data1.csv
- data2.csv
Using the following parameters:
- pattern = ‘dataset’
- include_extension_in_pattern = True
The renamed files will become:
- dataset_1.csv
- dataset_2.csv
Additional Examples:
- Using Different Starting Index:
bulk_rename_files(directory_path, “logfile”, start_index=100)
- Files will be renamed like logfile_100.txt, logfile_101.txt, etc.
- Renaming Files to a Different Extension:
bulk_rename_files(directory_path, “audio”, extension=”.mp3″)
- Files will be renamed like audio_1.mp3, audio_2.mp3, even if they were .wav before.
This approach provides flexibility for different types of file-renaming needs in bulk.
Leave a Reply