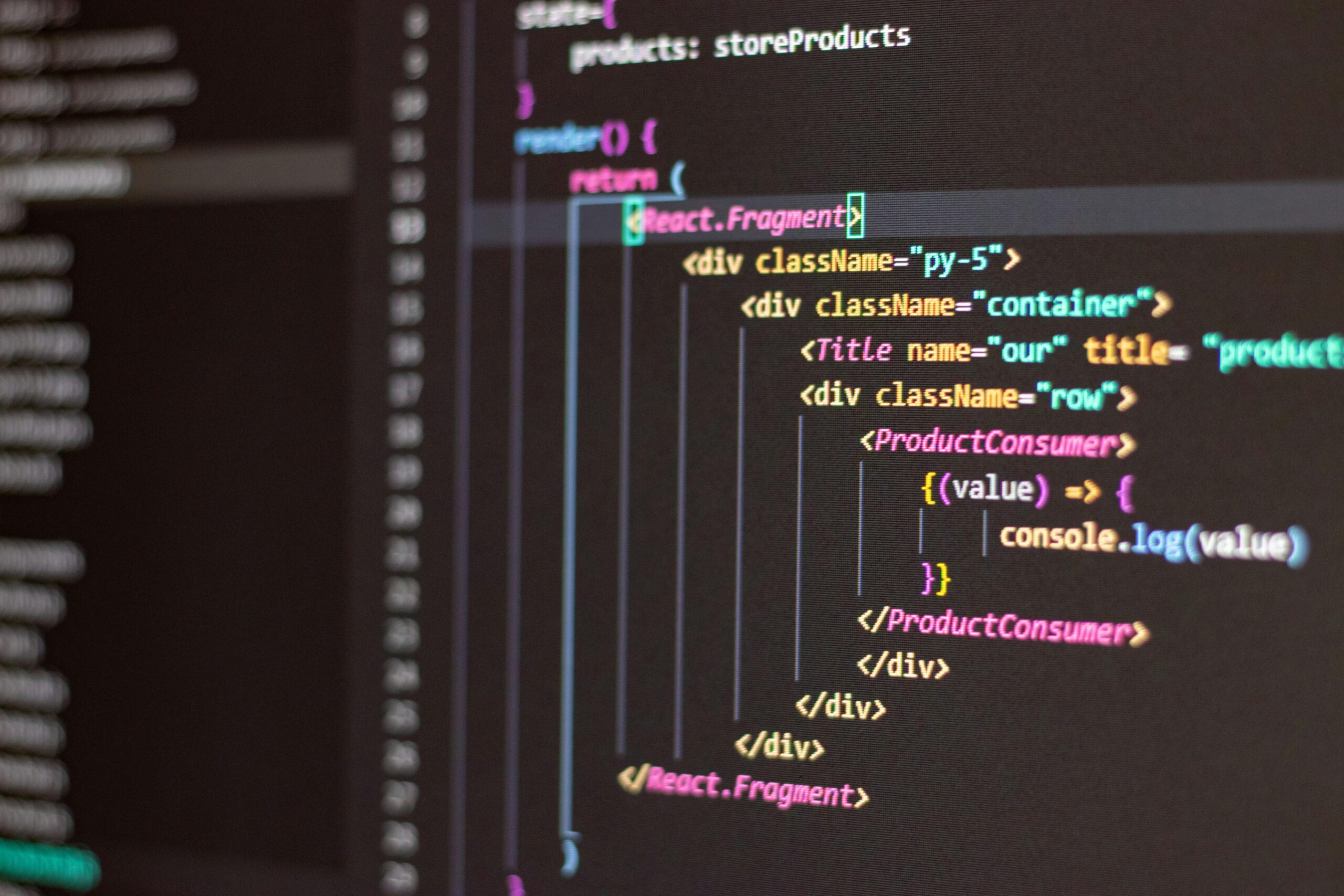
Photo by Rodrigo Santos
Swapping two numbers in Python is quite simple and can be done in a few different ways. Here are a few methods you can use:
1. Using a Temporary Variable
This is the traditional method:
# Define two numbers
a = 5
b = 10
# Print original values
print("Before swap: a =", a, "b =", b)
# Use a temporary variable to swap
temp = a
a = b
b = temp
# Print swapped values
print("After swap: a =", a, "b =", b)
2. Using Tuple Unpacking
This is a more Pythonic way:
# Define two numbers
a = 5
b = 10
# Print original values
print("Before swap: a =", a, "b =", b)
# Swap using tuple unpacking
a, b = b, a
# Print swapped values
print("After swap: a =", a, "b =", b)
3. Using Arithmetic Operations
This method avoids using a temporary variable but can be less readable:
# Define two numbers
a = 5
b = 10
# Print original values
print("Before swap: a =", a, "b =", b)
# Swap using arithmetic operations
a = a + b
b = a - b
a = a - b
# Print swapped values
print("After swap: a =", a, "b =", b)
4. Using XOR Bitwise Operator
This method is a bit more advanced and uses bitwise operations:
# Define two numbers
a = 5
b = 10
# Print original values
print("Before swap: a =", a, "b =", b)
# Swap using XOR
a = a ^ b
b = a ^ b
a = a ^ b
# Print swapped values
print("After swap: a =", a, "b =", b)
The tuple unpacking method is often preferred for its clarity and simplicity in Python.
Leave a Reply