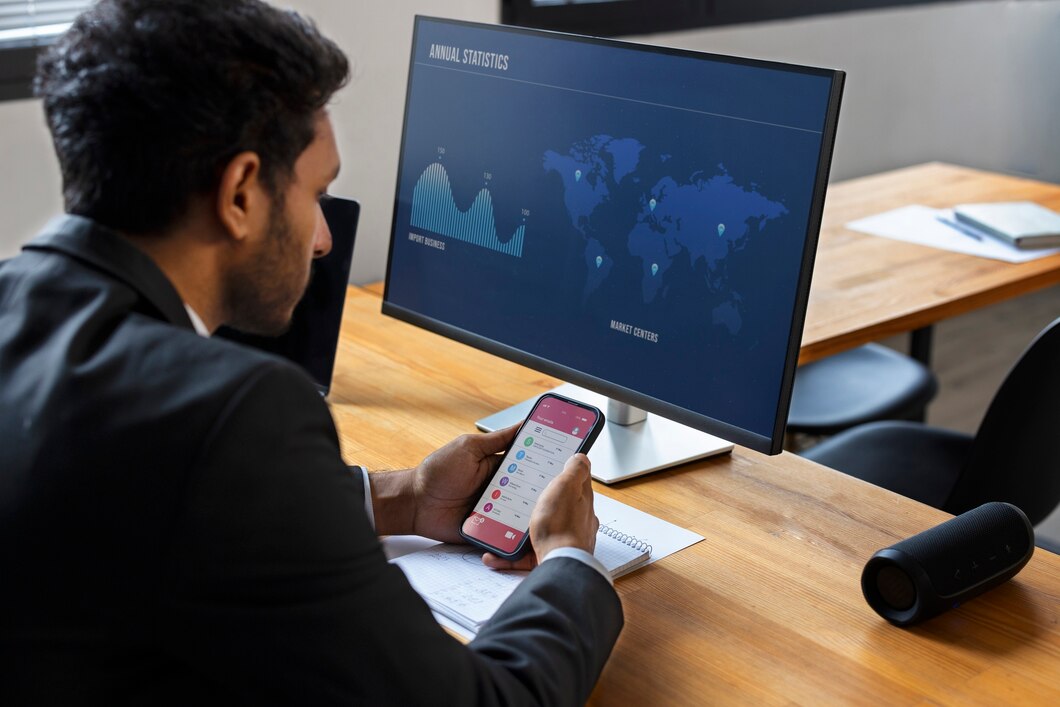
Photo by freepik
Creating a weather forecasting app using Flask and an external API like OpenWeatherMap or WeatherAPI is a great way to work with APIs and Flask web development. Here’s a step-by-step guide to help you build the app.
Prerequisites
Before starting, ensure you have the following installed:
- Python (preferably 3.7+)
- Flask (install using pip install flask)
- Requests (for making API calls, install using pip install requests)
- OpenWeatherMap API key (sign up for free at OpenWeatherMap to get an API key)
Step-by-Step Guide1. Set Up a Flask Project
First, create a directory for your project and navigate into it
mkdir weather_app
cd weather_app
First, create a directory for your project and navigate into it
pip install flask
Create a file app.py for the Flask server:
touch app.py
2. Create a Simple Flask Application
Let’s set up a basic Flask application. In app.py, define a simple route for the home page:
# app.py
from flask import Flask, render_template, request
import requests
app = Flask(__name__)
@app.route('/')
def index():
return render_template('index.html')
if __name__ == '__main__':
app.run(debug=True)
3. Get an API Key from OpenWeatherMap
- Go to OpenWeatherMap and sign up to create an account.
- Once you have an account, go to the API keys section and generate a new API key.
For this example, assume the API key is your_api_key.
4. Create a Function to Fetch Weather Data
Now, we need a function that fetches weather data from OpenWeatherMap using the city name input by the user.
Add the following function to app.py to make API requests:
# app.py
@app.route('/weather', methods=['POST'])
def weather():
city = request.form.get('city')
api_key = 'your_api_key' # Replace with your actual OpenWeatherMap API key
api_url = f'http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}&units=metric'
response = requests.get(api_url)
if response.status_code == 200:
data = response.json()
weather_data = {
'city': data['name'],
'temperature': data['main']['temp'],
'description': data['weather'][0]['description'],
'icon': data['weather'][0]['icon'],
}
return render_template('weather.html', weather_data=weather_data)
else:
error_message = "City not found. Please try again."
return render_template('index.html', error_message=error_message)
Here, the user submits a city name via an HTML form, and we fetch the current weather information by making a request to OpenWeatherMap API. If the city is found, it will render the weather data; otherwise, it will return an error message.
5. Create HTML Templates
Next, create the front-end of the app using HTML templates.
Create a templates directory and add two files: index.html for the homepage and weather.html to display the weather data.
index.html
<!-- templates/index.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Weather App</title>
</head>
<body>
<h1>Weather Forecast</h1>
<form method="POST" action="/weather">
<label for="city">Enter city name:</label>
<input type="text" id="city" name="city" required>
<button type="submit">Get Weather</button>
</form>
{% if error_message %}
<p style="color: red;">{{ error_message }}</p>
{% endif %}
</body>
</html>
weather.html
<!-- templates/weather.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Weather in {{ weather_data.city }}</title>
</head>
<body>
<h1>Weather in {{ weather_data.city }}</h1>
<p>Temperature: {{ weather_data.temperature }}°C</p>
<p>Description: {{ weather_data.description }}</p>
<img src="http://openweathermap.org/img/w/{{ weather_data.icon }}.png" alt="Weather icon">
<br><br>
<a href="/">Search for another city</a>
</body>
</html>
6. Run the Flask Application
Now, run the application using:
python app.py
Then, open a browser and go to http://127.0.0.1:5000/. You should see a form to enter the city name, and once you submit it, the app will fetch and display the weather details.
Final app.py File
Here is the complete app.py file:
from flask import Flask, render_template, request
import requests
app = Flask(__name__)
@app.route('/')
def index():
return render_template('index.html')
@app.route('/weather', methods=['POST'])
def weather():
city = request.form.get('city')
api_key = 'your_api_key' # Replace with your actual OpenWeatherMap API key
api_url = f'http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}&units=metric'
response = requests.get(api_url)
if response.status_code == 200:
data = response.json()
weather_data = {
'city': data['name'],
'temperature': data['main']['temp'],
'description': data['weather'][0]['description'],
'icon': data['weather'][0]['icon'],
}
return render_template('weather.html', weather_data=weather_data)
else:
error_message = "City not found. Please try again."
return render_template('index.html', error_message=error_message)
if __name__ == '__main__':
app.run(debug=True)
Conclusion
You now have a fully functional weather forecasting app built using Flask and the OpenWeatherMap API. You can extend this app further by:
- Adding more weather data (humidity, wind speed, etc.).
- Handling more errors (like API key issues).
- Adding CSS for better styling.
Leave a Reply