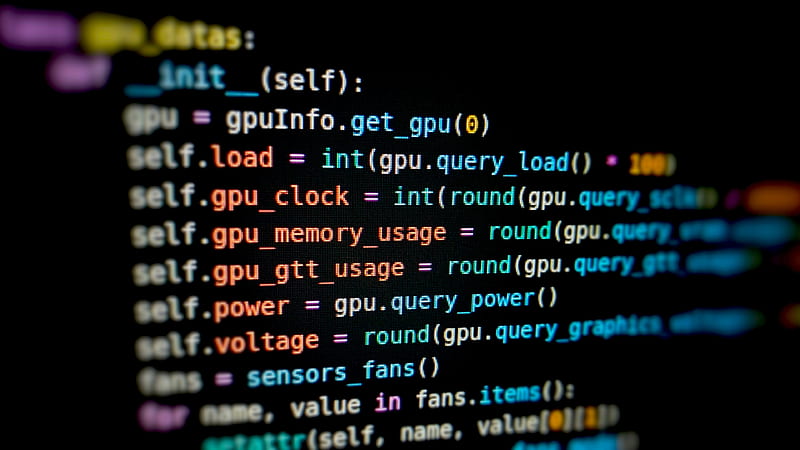
Photo by DICS
To write velocity data using Tecplot’s Python API (tecplot.py), you’ll typically want to create or modify a dataset and then write that dataset to a Tecplot format file. Below is a step-by-step guide to achieving this.
Step 1: Import the Tecplot API First, ensure that you have Tecplot 360 installed and the tecplot.py API available.
import tecplot as tp
from tecplot.constant import *
import numpy as np
Step 2: Create a New Dataset
You can create a new dataset that includes variables for the velocity components (e.g., U, V, W for 3D velocity vectors).
# Start a new Tecplot session
tp.session.connect()
# Create a new dataset with velocity components
dataset = tp.active_frame().create_dataset('Velocity Data', ['X', 'Y', 'Z', 'U', 'V', 'W'])
# Add a zone to the dataset (assuming structured grid)
zone = dataset.add_ordered_zone('Velocity Zone', (10, 10, 10))
# Generate some example data for the zone
x = np.linspace(0, 1, 10)
y = np.linspace(0, 1, 10)
z = np.linspace(0, 1, 10)
X, Y, Z = np.meshgrid(x, y, z, indexing='ij')
U = np.sin(2 * np.pi * X) * np.cos(2 * np.pi * Y) * np.sin(2 * np.pi * Z)
V = -np.cos(2 * np.pi * X) * np.sin(2 * np.pi * Y) * np.sin(2 * np.pi * Z)
W = np.cos(2 * np.pi * X) * np.cos(2 * np.pi * Y) * np.sin(2 * np.pi * Z)
# Assign the data to the dataset
zone.values('X')[:] = X.ravel()
zone.values('Y')[:] = Y.ravel()
zone.values('Z')[:] = Z.ravel()
zone.values('U')[:] = U.ravel()
zone.values('V')[:] = V.ravel()
zone.values('W')[:] = W.ravel()
Step 3: Save the Dataset
You can then write the dataset to a Tecplot file format (.plt).
# Save the dataset to a Tecplot file
tp.data.save_tecplot_ascii('velocity_data.dat', include_text=True, include_geom=True, zone_names=None)
tp.data.save_tecplot_plt('velocity_data.plt')
Step 4: Visualize the Data (Optional)
You can also create a simple plot to visualize the velocity vectors.
# Set the plot type to 3D Cartesian
tp.active_frame().plot_type = PlotType.Cartesian3D
# Get the active plot
plot = tp.active_frame().plot()
# Set the vector variables
plot.vector.u_variable = dataset.variable('U')
plot.vector.v_variable = dataset.variable('V')
plot.vector.w_variable = dataset.variable('W')
# Draw vectors
plot.show_vectors = True
# Save the plot as an image (optional)
tp.export.save_png('velocity_plot.png', 600)
# Disconnect the session
tp.session.disconnect()
Summary
- Import Tecplot and Create Dataset: You start by creating a dataset with variables for velocity components.
- Generate and Assign Data: Generate data for each velocity component and assign it to the dataset.
- Save the Dataset: Write the dataset to a Tecplot file (.plt or .dat).
- Optional Visualization: You can also visualize the velocity data and save it as an image.
This will allow you to use Tecplot’s powerful visualization tools to analyze the velocity data you generated or processed in Python.
Leave a Reply